Chapter 4: Deep Dive into Operators in C
Operators in C
Operators are symbols in C programming that perform various operations on operands (variables, constants, or expressions). They allow you to manipulate and perform calculations on data. Here are some commonly used operators in C:
- Arithmetic Operators.
- Assignment Operators.
- Relational Operators.
- Logical Operators.
- Bitwise Operators.
- Increment and Decrement Operators.
- Conditional (Ternary) Operator.
1. Arithmetic Operators:
- Why? Arithmetic operators allow you to perform mathematical calculations, making your programs more dynamic and flexible.
- When? You can use arithmetic operators when you need to add, subtract, multiply, divide, or find the remainder between two values.
- How? For example, you can use the addition operator (+) to add two numbers together.
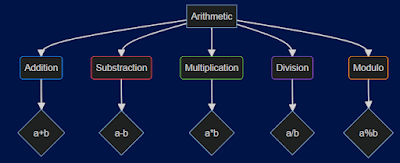
Arithmetic Operators
- Addition (+): Adds two operands together.
- Subtraction (-): Subtracts the second operand from the first.
- Multiplication (*): Multiplies two operands.
- Division (/): Divides the first operand by the second.
- Modulo (%): Returns the remainder of the division between the first operand and the second.
Have look at below example:
int num1 = 10, num2 = 4;
int sum = num1 + num2;
printf("Sum: %d\n", sum); // -> 14
int difference = num1 - num2;
printf("Difference: %d\n", difference); // -> 6
int product = num1 * num2;
printf("Product: %d\n", product); // -> 40
int quotient = num1 / num2;
printf("Quotient: %d\n", quotient); // -> 2
int remainder = num1 % num2;
printf("Remainder: %d\n", remainder); // -> 2
You can test above code live output, click Compile and see output2. Assignment Operators:
- Why? Assignment operators are essential for storing and manipulating data within a program. They allow you to assign values to variables, making it possible to perform calculations, update values, and store results.
- When? You can use assignment operators whenever you need to assign a value to a variable. This can be during variable initialization, updating variable values based on calculations or conditions, and modifying variables during program execution.
- How? To use an assignment operator, you need to specify the variable on the left-hand side of the operator and the value or expression on the right-hand side. The operator assigns the value on the right-hand side to the variable on the left-hand side.

Assignment Operators
- Assignment (=): Assigns the value on the right-hand side to the variable on the left-hand side. For example, x = 5; assigns the value 5 to the variable x.
- Compound assignment operators (+=, -=, *=, /=, %=): These operators perform an operation and assign the result to the variable. They are shorthand notations for performing the operation and assignment in a single step. For example:
- x += 5; is equivalent to x = x + 5; and adds 5 to the value of x and assigns the result back to x.
- y -= 3; is equivalent to y = y - 3; and subtracts 3 from the value of y and assigns the result back to y.
- z *= 2; is equivalent to z = z * 2; and multiplies the value of z by 2 and assigns the result back to z.
- a /= 4; is equivalent to a = a / 4; and divides the value of a by 4 and assigns the result back to a.
- b %= 7; is equivalent to b = b % 7; and performs the modulo operation on the value of b with 7 and assigns the remainder back to b.
Have look at below example:
int x = 10;
// Basic Assignment Operator (=)
int y = x;
printf("y = %d\n", y); // Output: y = 10
// Compound Assignment Operators
x += 5;
printf("x += 5: x = %d\n", x); // Output: x += 5: x = 15
x -= 3;
printf("x -= 3: x = %d\n", x); // Output: x -= 3: x = 12
x *= 2;
printf("x *= 2: x = %d\n", x); // Output: x *= 2: x = 24
x /= 4;
printf("x /= 4: x = %d\n", x); // Output: x /= 4: x = 6
x %= 5;
printf("x %%= 5: x = %d\n", x); // Output: x %= 5: x = 1
You can test above code live output, click Compile and see output3. Relational Operators:
- Why? Relational operators are used to compare values and conditions in C programming. They are essential for making decisions, controlling program flow, and creating conditional expressions.
- When? Relational operators are commonly used in situations where you need to compare values or conditions, such as in conditional statements (if-else, while, for), looping constructs, and logical expressions.
- How? Relational operators compare two operands and evaluate to a Boolean result (1 or 0) based on the specified condition. Here are the relational operators in C:

Relational Operators
- Why? Arithmetic operators allow you to perform mathematical calculations, making your programs more dynamic and flexible.
- When? You can use arithmetic operators when you need to add, subtract, multiply, divide, or find the remainder between two values.
- How? For example, you can use the addition operator (+) to add two numbers together.
![]() |
Arithmetic Operators |
- Addition (+): Adds two operands together.
- Subtraction (-): Subtracts the second operand from the first.
- Multiplication (*): Multiplies two operands.
- Division (/): Divides the first operand by the second.
- Modulo (%): Returns the remainder of the division between the first operand and the second.
int num1 = 10, num2 = 4; int sum = num1 + num2; printf("Sum: %d\n", sum); // -> 14 int difference = num1 - num2; printf("Difference: %d\n", difference); // -> 6 int product = num1 * num2; printf("Product: %d\n", product); // -> 40 int quotient = num1 / num2; printf("Quotient: %d\n", quotient); // -> 2 int remainder = num1 % num2; printf("Remainder: %d\n", remainder); // -> 2
You can test above code live output, click Compile and see output
2. Assignment Operators:
- Why? Assignment operators are essential for storing and manipulating data within a program. They allow you to assign values to variables, making it possible to perform calculations, update values, and store results.
- When? You can use assignment operators whenever you need to assign a value to a variable. This can be during variable initialization, updating variable values based on calculations or conditions, and modifying variables during program execution.
- How? To use an assignment operator, you need to specify the variable on the left-hand side of the operator and the value or expression on the right-hand side. The operator assigns the value on the right-hand side to the variable on the left-hand side.
![]() |
Assignment Operators |
- Assignment (=): Assigns the value on the right-hand side to the variable on the left-hand side. For example, x = 5; assigns the value 5 to the variable x.
- Compound assignment operators (+=, -=, *=, /=, %=): These operators perform an operation and assign the result to the variable. They are shorthand notations for performing the operation and assignment in a single step. For example:
- x += 5; is equivalent to x = x + 5; and adds 5 to the value of x and assigns the result back to x.
- y -= 3; is equivalent to y = y - 3; and subtracts 3 from the value of y and assigns the result back to y.
- z *= 2; is equivalent to z = z * 2; and multiplies the value of z by 2 and assigns the result back to z.
- a /= 4; is equivalent to a = a / 4; and divides the value of a by 4 and assigns the result back to a.
- b %= 7; is equivalent to b = b % 7; and performs the modulo operation on the value of b with 7 and assigns the remainder back to b.
int x = 10; // Basic Assignment Operator (=) int y = x; printf("y = %d\n", y); // Output: y = 10 // Compound Assignment Operators x += 5; printf("x += 5: x = %d\n", x); // Output: x += 5: x = 15 x -= 3; printf("x -= 3: x = %d\n", x); // Output: x -= 3: x = 12 x *= 2; printf("x *= 2: x = %d\n", x); // Output: x *= 2: x = 24 x /= 4; printf("x /= 4: x = %d\n", x); // Output: x /= 4: x = 6 x %= 5; printf("x %%= 5: x = %d\n", x); // Output: x %= 5: x = 1
You can test above code live output, click Compile and see output
3. Relational Operators:
- Why? Relational operators are used to compare values and conditions in C programming. They are essential for making decisions, controlling program flow, and creating conditional expressions.
- When? Relational operators are commonly used in situations where you need to compare values or conditions, such as in conditional statements (if-else, while, for), looping constructs, and logical expressions.
- How? Relational operators compare two operands and evaluate to a Boolean result (1 or 0) based on the specified condition. Here are the relational operators in C:
![]() |
Relational Operators |
- Equal to (==): Checks if two operands are equal. Example: 5 == 5 (result: true) Explanation: This statement checks if the value on the left (5) is equal to the value on the right (5). Since they are the same, the result is true.
- Not equal to (!=): Checks if two operands are not equal. Example: 10 != 7 (result: true)
Explanation: This statement checks if the value on the left (10) is not equal to the value on the right (7). Since they are different, the result is true.
- Greater than (>): Checks if the left operand is greater than the right operand. Example: 8 > 3 (result: true)
Explanation: This statement checks if the value on the left (8) is greater than the value on the right (3). Since 8 is indeed greater than 3, the result is true.
- Less than (<): Checks if the left operand is less than the right operand. Example: 2 < 6 (result: true)
Explanation: This statement checks if the value on the left (2) is less than the value on the right (6). Since 2 is indeed less than 6, the result is true.
- Greater than or equal to (>=): Checks if the left operand is greater than or equal to the right operand. Example: 5 >= 5 (result: true)
Explanation:: This statement checks if the value on the left (5) is greater than or equal to the value on the right (5). Since 5 is equal to 5, the result is true.
- Less than or equal to (<=): Checks if the left operand is less than or equal to the right operand. Example: 3 <= 2 (result: false)
Explanation: This statement checks if the value on the left (3) is less than or equal to the value on the right (2). Since 3 is not less than or equal to 2, the result is false.
Have look at below example:
int num1 = 10;
int num2 = 5;
// Equal to (==)
if (num1 == num2) {
printf("%d is equal to %d\n", num1, num2);
} else {
printf("%d is not equal to %d\n", num1, num2); // Result: 10 is not equal to 5
}
// Not equal to (!=)
if (num1 != num2) {
printf("%d is not equal to %d\n", num1, num2); // Result: 10 is not equal to 5
} else {
printf("%d is equal to %d\n", num1, num2);
}
// Greater than (>)
if (num1 > num2) {
printf("%d is greater than %d\n", num1, num2); // Result: 10 is greater than 5
} else {
printf("%d is not greater than %d\n", num1, num2);
}
// Less than (<)
if (num1 < num2) {
printf("%d is less than %d\n", num1, num2);
} else {
printf("%d is not less than %d\n", num1, num2); // Result: 10 is not less than 5
}
// Greater than or equal to (>=)
if (num1 >= num2) {
printf("%d is greater than or equal to %d\n", num1, num2); // Result: 10 is greater than or equal to 5
} else {
printf("%d is not greater than or equal to %d\n", num1, num2);
}
// Less than or equal to (<=)
if (num1 <= num2) {
printf("%d is less than or equal to %d\n", num1, num2);
} else {
printf("%d is not less than or equal to %d\n", num1, num2); // Result: 10 is not less than or equal to 5
}
You can test above code live output, click Compile and see output
- Equal to (==): Checks if two operands are equal. Example: 5 == 5 (result: true) Explanation: This statement checks if the value on the left (5) is equal to the value on the right (5). Since they are the same, the result is true.
- Not equal to (!=): Checks if two operands are not equal. Example: 10 != 7 (result: true)
Explanation: This statement checks if the value on the left (10) is not equal to the value on the right (7). Since they are different, the result is true.
- Greater than (>): Checks if the left operand is greater than the right operand. Example: 8 > 3 (result: true)
Explanation: This statement checks if the value on the left (8) is greater than the value on the right (3). Since 8 is indeed greater than 3, the result is true.
- Less than (<): Checks if the left operand is less than the right operand. Example: 2 < 6 (result: true)
Explanation: This statement checks if the value on the left (2) is less than the value on the right (6). Since 2 is indeed less than 6, the result is true.
- Greater than or equal to (>=): Checks if the left operand is greater than or equal to the right operand. Example: 5 >= 5 (result: true)
Explanation:: This statement checks if the value on the left (5) is greater than or equal to the value on the right (5). Since 5 is equal to 5, the result is true.
- Less than or equal to (<=): Checks if the left operand is less than or equal to the right operand. Example: 3 <= 2 (result: false)
Explanation: This statement checks if the value on the left (3) is less than or equal to the value on the right (2). Since 3 is not less than or equal to 2, the result is false.
int num1 = 10; int num2 = 5; // Equal to (==) if (num1 == num2) { printf("%d is equal to %d\n", num1, num2); } else { printf("%d is not equal to %d\n", num1, num2); // Result: 10 is not equal to 5 } // Not equal to (!=) if (num1 != num2) { printf("%d is not equal to %d\n", num1, num2); // Result: 10 is not equal to 5 } else { printf("%d is equal to %d\n", num1, num2); } // Greater than (>) if (num1 > num2) { printf("%d is greater than %d\n", num1, num2); // Result: 10 is greater than 5 } else { printf("%d is not greater than %d\n", num1, num2); } // Less than (<) if (num1 < num2) { printf("%d is less than %d\n", num1, num2); } else { printf("%d is not less than %d\n", num1, num2); // Result: 10 is not less than 5 } // Greater than or equal to (>=) if (num1 >= num2) { printf("%d is greater than or equal to %d\n", num1, num2); // Result: 10 is greater than or equal to 5 } else { printf("%d is not greater than or equal to %d\n", num1, num2); } // Less than or equal to (<=) if (num1 <= num2) { printf("%d is less than or equal to %d\n", num1, num2); } else { printf("%d is not less than or equal to %d\n", num1, num2); // Result: 10 is not less than or equal to 5 }
You can test above code live output, click Compile and see output
4. Logical Operators:
Logical Operators in C are used to perform logical operations on Boolean values (true or false) or expressions. They allow you to combine multiple conditions and evaluate the overall result.
Why ? Logical operators are used to make decisions, control program flow, and perform conditional operations based on multiple conditions. They are particularly useful when you need to combine multiple conditions or check for the validity of certain expressions.
When ? Logical operators are commonly used in situations where you need to evaluate multiple conditions and make decisions based on their combined results. They are frequently used in if-else statements, while loops, and for loops to control the flow of a program based on specific conditions.
How ? In C, there are three logical operators: AND (&&), OR (||), and NOT (!).: condition1 && condition2,
condition1 || condition2, !condition etc.

Logical Operators
- AND Operator (&&): The AND operator returns true if both the operands are true; otherwise, it returns false. Example: result = (x > 5 && y < 10);
Explanation: The result variable will be assigned the value true if both conditions (x > 5) and (y < 10) are true; otherwise, it will be assigned the value false.
- OR Operator (||): The OR operator returns true if at least one of the operands is true; otherwise, it returns false. Example: result = (x > 5 || y < 10);
Explanation: The result variable will be assigned the value true if either condition (x > 5) or condition (y < 10) is true; otherwise, it will be assigned the value false.
- NOT Operator (!): The NOT operator negates the value of a condition. It returns true if the condition is false and false if the condition is true. Example: result = !(x > 5);
Explanation: The result variable will be assigned the value true if the condition (x > 5) is false; otherwise, it will be assigned the value false.
Have look at below example:
int x = 7;
int y = 3;
int result;
// AND Operator (&&)
result = (x > 5 && y < 10); // Result: true (both conditions are true)
// OR Operator (||)
result = (x > 5 || y < 10); // Result: true (at least one condition is true)
// NOT Operator (!)
result = !(x > 5); // Result: false (negation of the condition)
return 0;
You can test above code live output, click Compile and see output
Logical Operators in C are used to perform logical operations on Boolean values (true or false) or expressions. They allow you to combine multiple conditions and evaluate the overall result.
Why ? Logical operators are used to make decisions, control program flow, and perform conditional operations based on multiple conditions. They are particularly useful when you need to combine multiple conditions or check for the validity of certain expressions.
When ? Logical operators are commonly used in situations where you need to evaluate multiple conditions and make decisions based on their combined results. They are frequently used in if-else statements, while loops, and for loops to control the flow of a program based on specific conditions.
How ? In C, there are three logical operators: AND (&&), OR (||), and NOT (!).
: condition1 && condition2,
condition1 || condition2, !condition etc.
![]() |
Logical Operators |
- AND Operator (&&): The AND operator returns true if both the operands are true; otherwise, it returns false. Example: result = (x > 5 && y < 10);
Explanation: The result variable will be assigned the value true if both conditions (x > 5) and (y < 10) are true; otherwise, it will be assigned the value false.
- OR Operator (||): The OR operator returns true if at least one of the operands is true; otherwise, it returns false. Example: result = (x > 5 || y < 10);
Explanation: The result variable will be assigned the value true if either condition (x > 5) or condition (y < 10) is true; otherwise, it will be assigned the value false.
- NOT Operator (!): The NOT operator negates the value of a condition. It returns true if the condition is false and false if the condition is true. Example: result = !(x > 5);
Explanation: The result variable will be assigned the value true if the condition (x > 5) is false; otherwise, it will be assigned the value false.
Have look at below example:
int x = 7; int y = 3; int result; // AND Operator (&&) result = (x > 5 && y < 10); // Result: true (both conditions are true) // OR Operator (||) result = (x > 5 || y < 10); // Result: true (at least one condition is true) // NOT Operator (!) result = !(x > 5); // Result: false (negation of the condition) return 0;
You can test above code live output, click Compile and see output
5. Bitwise Operators:
Bitwise operators in C are used to perform operations on individual bits of integer values. They manipulate the binary representation of the values at the bit level. Here's an explanation of bitwise operators
Why ? Bitwise operators are primarily used for low-level programming, such as dealing with hardware devices, manipulating bits for specific operations, and optimizing memory usage. They provide a way to perform operations at the binary level, which can be useful in certain scenarios.
When ? You can use bitwise operators when you need to manipulate individual bits within an integer value. This can be useful in situations where you want to set, clear, or toggle specific bits, extract or insert bit patterns, or perform bitwise logical operations.
How ? In C, there are six bitwise operators: AND (&), OR (|), XOR (^), NOT (~), left shift (<<), and right shift (>>).
- Bitwise AND (&): The bitwise AND operator performs a bitwise AND operation on each pair of corresponding bits. It compares the bits of two operands and produces a new value where each bit is set to 1 only if both corresponding bits are 1. Otherwise, it sets the bit to 0.
Example:
12 & 10 results in 8
Binary: 0000110 & 00001010 = 00001000
- Bitwise OR (|):The bitwise OR operator performs a bitwise OR operation on each pair of corresponding bits. It compares the bits of two operands and produces a new value where each bit is set to 1 if at least one of the corresponding bits is 1. Otherwise, it sets the bit to 0.
Example: 12 | 10 results in 14
Binary: 00001110 | 00001010 = 00001110
- Bitwise XOR (^): The bitwise XOR operator performs a bitwise XOR (exclusive OR) operation on each pair of corresponding bits. It compares the bits of two operands and produces a new value where each bit is set to 1 if the corresponding bits are different. Otherwise, it sets the bit to 0
Example: 12 ^ 10 results in 6
Binary: 00001100 ^ 00001010 = 00000110
- Left Shift (<<):The left shift operator shifts the bits of the left operand to the left by a specified number of positions. It effectively multiplies the left operand by 2 raised to the power of the number of positions shifted.
Example: 12 << 2 results in 48
Binary: 00001100 << 2 = 00110000
- Right Shift (>>):The right shift operator shifts the bits of the left operand to the right by a specified number of positions. It effectively divides the left operand by 2 raised to the power of the number of positions shifted.
Example: 12 >> 2 results in 3
Binary: 00001100 >> 2 = 00000011
- Bitwise Complement (~):The bitwise complement operator flips each bit of the operand. It changes each 0 to 1 and each 1 to 0.
Example: ~12 results in -13 (two's complement representation)
Binary: ~00001100 = 11110011
Have look at below example: unsigned int a = 10; // Binary: 00001010
unsigned int b = 6; // Binary: 00000110
unsigned int result;
result = a & b; // Bitwise AND
printf("a & b = %u\n", result); // Output: 2 (Binary: 00000010)
result = a | b; // Bitwise OR
printf("a | b = %u\n", result); // Output: 14 (Binary: 00001110)
result = a ^ b; // Bitwise XOR
printf("a ^ b = %u\n", result); // Output: 12 (Binary: 00001100)
result = a << 2; // Left Shift by 2 positions
printf("a << 2 = %u\n", result); // Output: 40 (Binary: 00101000)
result = b >> 1; // Right Shift by 1 position
printf("b >> 1 = %u\n", result); // Output: 3 (Binary: 00000011)
result = ~a; // Bitwise Complement
printf("~a = %u\n", result); // Output: 4294967285 (Binary: 11111111111111111111111111110101)
You can test above code live output, click Compile and see output
Bitwise operators in C are used to perform operations on individual bits of integer values. They manipulate the binary representation of the values at the bit level. Here's an explanation of bitwise operators
Why ? Bitwise operators are primarily used for low-level programming, such as dealing with hardware devices, manipulating bits for specific operations, and optimizing memory usage. They provide a way to perform operations at the binary level, which can be useful in certain scenarios.
When ? You can use bitwise operators when you need to manipulate individual bits within an integer value. This can be useful in situations where you want to set, clear, or toggle specific bits, extract or insert bit patterns, or perform bitwise logical operations.
How ? In C, there are six bitwise operators: AND (&), OR (|), XOR (^), NOT (~), left shift (<<), and right shift (>>).
- Bitwise AND (&): The bitwise AND operator performs a bitwise AND operation on each pair of corresponding bits. It compares the bits of two operands and produces a new value where each bit is set to 1 only if both corresponding bits are 1. Otherwise, it sets the bit to 0.
Example: 12 & 10 results in 8
Binary: 0000110 & 00001010 = 00001000
- Bitwise OR (|):The bitwise OR operator performs a bitwise OR operation on each pair of corresponding bits. It compares the bits of two operands and produces a new value where each bit is set to 1 if at least one of the corresponding bits is 1. Otherwise, it sets the bit to 0.
Example: 12 | 10 results in 14
Binary: 00001110 | 00001010 = 00001110 - Bitwise XOR (^): The bitwise XOR operator performs a bitwise XOR (exclusive OR) operation on each pair of corresponding bits. It compares the bits of two operands and produces a new value where each bit is set to 1 if the corresponding bits are different. Otherwise, it sets the bit to 0
Example: 12 ^ 10 results in 6
Binary: 00001100 ^ 00001010 = 00000110
- Left Shift (<<):The left shift operator shifts the bits of the left operand to the left by a specified number of positions. It effectively multiplies the left operand by 2 raised to the power of the number of positions shifted.
Example: 12 << 2 results in 48
Binary: 00001100 << 2 = 00110000
- Right Shift (>>):The right shift operator shifts the bits of the left operand to the right by a specified number of positions. It effectively divides the left operand by 2 raised to the power of the number of positions shifted.
Example: 12 >> 2 results in 3
Binary: 00001100 >> 2 = 00000011
- Bitwise Complement (~):The bitwise complement operator flips each bit of the operand. It changes each 0 to 1 and each 1 to 0.
Example: ~12 results in -13 (two's complement representation)
Binary: ~00001100 = 11110011
Have look at below example:
unsigned int a = 10; // Binary: 00001010 unsigned int b = 6; // Binary: 00000110 unsigned int result; result = a & b; // Bitwise AND printf("a & b = %u\n", result); // Output: 2 (Binary: 00000010) result = a | b; // Bitwise OR printf("a | b = %u\n", result); // Output: 14 (Binary: 00001110) result = a ^ b; // Bitwise XOR printf("a ^ b = %u\n", result); // Output: 12 (Binary: 00001100) result = a << 2; // Left Shift by 2 positions printf("a << 2 = %u\n", result); // Output: 40 (Binary: 00101000) result = b >> 1; // Right Shift by 1 position printf("b >> 1 = %u\n", result); // Output: 3 (Binary: 00000011) result = ~a; // Bitwise Complement printf("~a = %u\n", result); // Output: 4294967285 (Binary: 11111111111111111111111111110101)
You can test above code live output, click Compile and see output
6. Increment & Decrement Operators:
Why ? The Increment & Decrement Operators are used to modify the value of variables conveniently and efficiently. They are commonly used in loops, calculations, and iterative operations. The operators provide a concise way to increment or decrement the value of a variable by a fixed amount.
When ? The Increment & Decrement Operators are used when you need to update the value of a variable by a specific amount, such as incrementing or decrementing by 1. They are particularly useful in situations like counting, iterating over arrays or loops, and implementing numerical operations.
How ? In C, the Increment Operator (++x or x++) increases the value of a variable by 1, while the Decrement Operator (--x or x--) decreases the value of a variable by 1. The operators can be used in both prefix and postfix forms:
Why ? The Increment & Decrement Operators are used to modify the value of variables conveniently and efficiently. They are commonly used in loops, calculations, and iterative operations. The operators provide a concise way to increment or decrement the value of a variable by a fixed amount.
When ? The Increment & Decrement Operators are used when you need to update the value of a variable by a specific amount, such as incrementing or decrementing by 1. They are particularly useful in situations like counting, iterating over arrays or loops, and implementing numerical operations.
How ? In C, the Increment Operator (++x or x++) increases the value of a variable by 1, while the Decrement Operator (--x or x--) decreases the value of a variable by 1. The operators can be used in both prefix and postfix forms:

Increment & Decrement Operators
- Prefix Increment (++): The variable is incremented first, and the updated value is used in the expression or assignment statement. It returns the new value after incrementing.
Example: x = 5; y = ++x;
Explanation: In this example, the value of x is incremented by 1 before it is assigned to y. So, after the operation, x becomes 6, and the value of y is also 6 because it receives the updated value of x. - Postfix Increment (x++): The current value of the variable is used in the expression or assignment statement, and then the variable is incremented. It returns the original value before incrementing.
Example: x = 5; y = x++;
Explanation: In this example, the current value of x (5) is used in the assignment statement to initialize y. After that, x is incremented by 1. So, after the operation, x becomes 6, but the value of y is 5 because it received the original value of x before the increment.
- Prefix Decrement (--): The variable is decremented first, and the updated value is used in the expression or assignment statement. It returns the new value after decrementing.
Example: x = 5; y = --x;
Explanation: In this example, the value of x is decremented by 1 before it is assigned to y. So, after the operation, x becomes 4, and the value of y is also 4 because it receives the updated value of x. - Postfix Decrement (x--): The current value of the variable is used in the expression or assignment statement, and then the variable is decremented. It returns the original value before decrementing.
Example: int x = 5; int y = x--;
Explanation: In this example, the current value of x (5) is used in the assignment statement to initialize y. After that, x is decremented by 1. So, after the operation, x becomes 4, but the value of y is 5 because it received the original value of x before the decrement.
Have look at below example:
int x = 5;
int y;
// Prefix Increment (++x)
y = ++x; // Increment x first, then assign to y
printf("Prefix Increment: y = ++x;\n");
printf("x: %d, y: %d\n", x, y); // Output: x: 6, y: 6
// Postfix Increment (x++)
y = x++; // Assign x to y first, then increment x
printf("Postfix Increment: y = x++;\n");
printf("x: %d, y: %d\n", x, y); // Output: x: 7, y: 6
// Prefix Decrement (--x)
y = --x; // Decrement x first, then assign to y
printf("Prefix Decrement: y = --x;\n");
printf("x: %d, y: %d\n", x, y); // Output: x: 6, y: 6
// Postfix Decrement (x--)
y = x--; // Assign x to y first, then decrement x
printf("Postfix Decrement: y = x--;\n");
printf("x: %d, y: %d\n", x, y); // Output: x: 5, y: 6
![]() |
Increment & Decrement Operators |
- Prefix Increment (++): The variable is incremented first, and the updated value is used in the expression or assignment statement. It returns the new value after incrementing.
Example: x = 5; y = ++x;
Explanation: In this example, the value of x is incremented by 1 before it is assigned to y. So, after the operation, x becomes 6, and the value of y is also 6 because it receives the updated value of x. - Postfix Increment (x++): The current value of the variable is used in the expression or assignment statement, and then the variable is incremented. It returns the original value before incrementing.
Example: x = 5; y = x++;
Explanation: In this example, the current value of x (5) is used in the assignment statement to initialize y. After that, x is incremented by 1. So, after the operation, x becomes 6, but the value of y is 5 because it received the original value of x before the increment.
- Prefix Decrement (--): The variable is decremented first, and the updated value is used in the expression or assignment statement. It returns the new value after decrementing.
Example: x = 5; y = --x;
Explanation: In this example, the value of x is decremented by 1 before it is assigned to y. So, after the operation, x becomes 4, and the value of y is also 4 because it receives the updated value of x. - Postfix Decrement (x--): The current value of the variable is used in the expression or assignment statement, and then the variable is decremented. It returns the original value before decrementing.
Example: int x = 5; int y = x--;
Explanation: In this example, the current value of x (5) is used in the assignment statement to initialize y. After that, x is decremented by 1. So, after the operation, x becomes 4, but the value of y is 5 because it received the original value of x before the decrement.
int x = 5; int y; // Prefix Increment (++x) y = ++x; // Increment x first, then assign to y printf("Prefix Increment: y = ++x;\n"); printf("x: %d, y: %d\n", x, y); // Output: x: 6, y: 6 // Postfix Increment (x++) y = x++; // Assign x to y first, then increment x printf("Postfix Increment: y = x++;\n"); printf("x: %d, y: %d\n", x, y); // Output: x: 7, y: 6 // Prefix Decrement (--x) y = --x; // Decrement x first, then assign to y printf("Prefix Decrement: y = --x;\n"); printf("x: %d, y: %d\n", x, y); // Output: x: 6, y: 6 // Postfix Decrement (x--) y = x--; // Assign x to y first, then decrement x printf("Postfix Decrement: y = x--;\n"); printf("x: %d, y: %d\n", x, y); // Output: x: 5, y: 6
You can test above code live output, click Compile and see output
7. Conditional (Ternary) Operators:
Why ? Conditional operators provide a compact and efficient way to express simple conditional statements. They can make your code more concise and readable by reducing the need for multiple if-else statements.When ? Conditional operators are useful when you have a simple condition and want to assign a value based on that condition. They are commonly used in situations where you need to make a decision based on a single condition without needing a full if-else structure.How ? The syntax of the conditional operator is condition ? expression1 : expression2.The condition is evaluated first. If it is true, expression1 is evaluated and becomes the result of the expression. Otherwise, expression2 is evaluated and becomes the result.The result of the conditional operator can be used in assignments or as part of an expression.

Ternary Operator
Have look at below example:
int a = 10;
int b = 5;
int max = (a > b) ? a : b; // If a is greater than b, assign a to max; otherwise, assign b to max
int age = 20;
const char* message = (age >= 18) ? "You are an adult" : "You are a minor"; // If age is greater than or equal to 18, assign "You are an adult"; otherwise, assign "You are a minor"
printf("Max: %d\n", max); // Output: Max: 10
printf("Message: %s\n", message); // Output: Message: You are an adult
You can test above code live output, click Compile and see output
Test your Skills in the next Post.
NOTE: Kindly provide feedback and comments below, which will help me improve the content. if any doubts or questions please comment below.
Why ? Conditional operators provide a compact and efficient way to express simple conditional statements. They can make your code more concise and readable by reducing the need for multiple if-else statements.
When ? Conditional operators are useful when you have a simple condition and want to assign a value based on that condition. They are commonly used in situations where you need to make a decision based on a single condition without needing a full if-else structure.
How ? The syntax of the conditional operator is condition ? expression1 : expression2.
The condition is evaluated first. If it is true, expression1 is evaluated and becomes the result of the expression. Otherwise, expression2 is evaluated and becomes the result.
The result of the conditional operator can be used in assignments or as part of an expression.
![]() |
Ternary Operator |
Have look at below example:
int a = 10; int b = 5; int max = (a > b) ? a : b; // If a is greater than b, assign a to max; otherwise, assign b to max int age = 20; const char* message = (age >= 18) ? "You are an adult" : "You are a minor"; // If age is greater than or equal to 18, assign "You are an adult"; otherwise, assign "You are a minor" printf("Max: %d\n", max); // Output: Max: 10 printf("Message: %s\n", message); // Output: Message: You are an adult
You can test above code live output, click Compile and see output
Test your Skills in the next Post.
NOTE: Kindly provide feedback and comments below, which will help me improve the content. if any doubts or questions please comment below.
Thank you for reading this blog post! To explore more chapters, click the button below:
Master C Programming Index
Comments
Post a Comment