Chapter 5: Tackling Typecasting, Decision Control in C
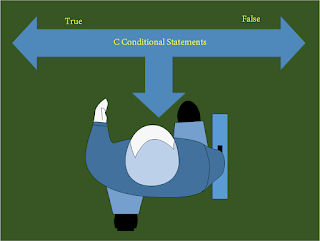
Decision Control Type Conversion Type conversion , also known as type coercion , is an automatic process performed by the compiler (or programming language) to convert one data type into another without explicit instructions from the programmer. The purpose of type conversion is to facilitate operations between different data types and ensure compatibility. For Example, Consider the code below: int num1 = 10 ; float num2 = 3.14 ; float sum = num1 + num2; The code declares three variables: num1 (an integer), num2 (a float), and sum (a float). The variable num1 is initialized with the value 10 , and num2 is initialized with 3.14 . The expression num1 + num2 performs addition between an integer and a float. During this operation, the compiler automatically converts the num1 integer value to a float. This conversion, also known as type coercion, enables us to add different data types together. The result of the addition is 13.14 , which is then stored in th